
Answer:
Check the explanation
Explanation:
//Define the class.
public class Loan implements java.io.Serializable {
//Define the variables.
private static final long serialVersionUID = 1L;
private double annualInterestRate;
private int numberOfYears;
private double loanAmount;
private java.util.Date loanDate;
//Define the default constructor.
public Loan() {
this(2.5, 1, 1000);
}
//Define the multi argument constructor.
protected Loan(double annualInterestRate, int numberOfYears,
double loanAmount) {
this.annualInterestRate = annualInterestRate;
this.numberOfYears = numberOfYears;
this.loanAmount = loanAmount;
loanDate = new java.util.Date();
}
//Define the getter and setter method.
public double getAnnualInterestRate() {
return annualInterestRate;
}
public void setAnnualInterestRate(double annualInterestRate) {
this.annualInterestRate = annualInterestRate;
}
public int getNumberOfYears() {
return numberOfYears;
}
public void setNumberOfYears(int numberOfYears) {
this.numberOfYears = numberOfYears;
}
public double getLoanAmount() {
return loanAmount;
}
public void setLoanAmount(double loanAmount) {
this.loanAmount = loanAmount;
}
//Define the method to compute the monthly payment.
public double getMonthlyPayment() {
double monthlyInterestRate = annualInterestRate / 1200;
double monthlyPayment = loanAmount * monthlyInterestRate / (1 -
(Math.pow(1 / (1 + monthlyInterestRate), numberOfYears * 12)));
return monthlyPayment;
}
//Define the method to get the total payment.
public double getTotalPayment() {
double totalPayment = getMonthlyPayment() * numberOfYears * 12;
return totalPayment;
}
public java.util.Date getLoanDate() {
return loanDate;
}
}
//Define the client class.
public class ClientLoan extends Application {
//Create the server object.
ServerLoan serverLoan;
//Declare the variables.
int y;
double r, a, mp=0, tp=0;
String result,d1;
//Create the button.
Button b = new Button("Submit");
//Define the method stage.
public void start(Stage primaryStage) throws Exception {
TimeZone.setDefault(TimeZone.getTimeZone("EST"));
TimeZone.getDefault();
d1 = "Server Started at " +new Date();
//Create the GUI.
Label l1=new Label("Annual Interest Rate");
Label l2 = new Label("Number Of Years:");
Label l3 = new Label("Loan Amount");
TextField t1=new TextField();
TextField t2=new TextField();
TextField t3=new TextField();
TextArea ta = new TextArea();
//Add the components in the gridpane.
GridPane root = new GridPane();
root.addRow(0, l1, t1);
root.addRow(1, l2, t2, b);
root.addRow(5,l3, t3);
root.addRow(6, ta);
//Add gridpane and text area to vbox.
VBox vb = new VBox(root, ta);
//Add vbox to the scene.
Scene scene=new Scene(vb,400,250);
//Add button click event.
b.setOnAction(value -> {
//Get the user input from the text field.
r = Double.parseDouble(t1.getText());
y = Integer.parseInt(t2.getText());
a = Double.parseDouble(t3.getText());
//Create the loan class object.
Loan obj = new Loan(r, y, a);
//Call the method to compute the results.
mp = obj.getMonthlyPayment();
tp = obj.getTotalPayment();
//Format the results.
result = "Annual Interest Rate: "+ r+"\n"+
"Number of Years: "+y+"\n"+
"Loan Amount: "+a+"\n"+
"monthlyPayment: "+mp+"\n"+
"totalPayment: "+tp;
//Add the result to the textarea.
ta.setText(result);
//Create an object of the server class.
serverLoan = new ServerLoan(this);
});
//Set the scene to the stage.
//Set the stage title.
//Make the scene visible.
primaryStage.setScene(scene);
primaryStage.setTitle("ClientLoan");
primaryStage.show();
}
//Define the main method lauch the application.
public static void main(String args[])
{
launch(args);
}
//Define the server class.
class ServerLoan extends Stage {
//Create the client loan object.
ClientLoan parent;
//Create the stage object.
Stage subStage;
//Create the text area.
TextArea ta = new TextArea();
//Define the constructor.
private ServerLoan(ClientLoan aThis) {
//Get the time in desired timezone.
TimeZone.setDefault(TimeZone.getTimeZone("EST"));
TimeZone.getDefault();
//Format the date with message.
String d2 = "Connected to client at " +new Date();
//Initialize the object.
parent = aThis;
//Add the date and the result to
//the text area.
ta.setText(d1);
ta.appendText("\n"+ d2);
ta.appendText("\n"+result);
//Create the grouppane.
GridPane root = new GridPane();
//Add text area to the group pane.
root.addRow(0, ta);
//Initialise the stage object.
subStage = new Stage();
//Add gridpane to the scene.
Scene scene = new Scene(root, 400, 200);
//Set the scene to the stage.
//Set the stage title.
//Make the scene visible.
subStage.setScene(scene);
subStage.setTitle("ServerLoan");
subStage.show();
}
}
}
Kindly check the Output in the attached image below.
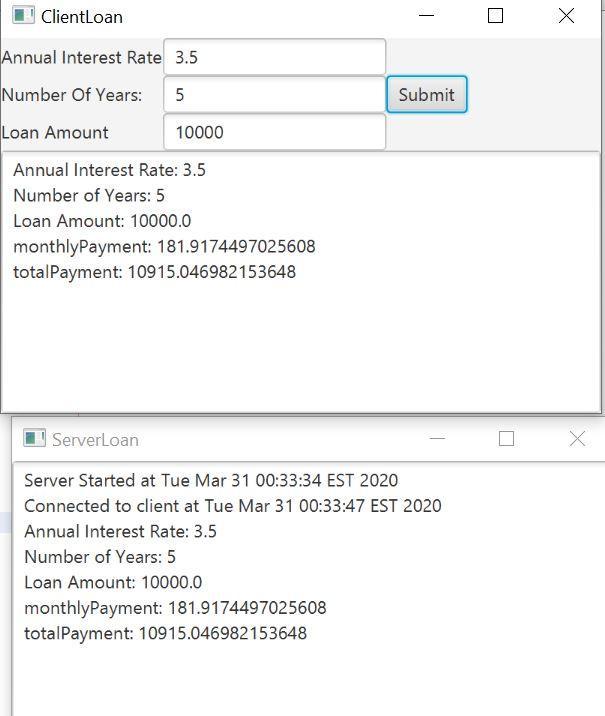